In this article we will learn how you can add event handlers to your react JSX.
Event handlers are functions that you can create. These functions will be triggered in response to a user interaction like : user clicking, hovering, focusing on inputs etc
Dead Simple Chat allows you to add chat in minutes to your React Application with the powerful JavaScript Chat SDK and API.
Pre-requisites
- Basic knowledge of JavaScript
- Basic knowledge of HTML
- Some knowledge of React
What are you going to learn in this article:
- What are the different ways a event handler can be written
- How event handling logic can be given to a child component from a parent component
- How events propogate through the app and how to stop them if needed
Let us start with the learning
Dead Simple Chat allows you to add chat in minutes to your React Application with the powerful JavaScript Chat SDK and API.
Step1: How to add event handlers to your code.
To add a event handler,
- define a function
- Pass it as a prop to the appropriate JSX tag
For example let us create a button that doesn't do anything then add a event handler to it.
export default function Button() {
return (
<button>
Clicking on the button doesn't do anything
</button>
);
}
Let us define a function to show an alert when the button is clicked. Here are the steps to do that
- Declare a function and name it handleClick inside the button component
- then write code inside the handleClick function to show an alert
- declare the
onClick={handleClick}
and pass it as a prop to the button.
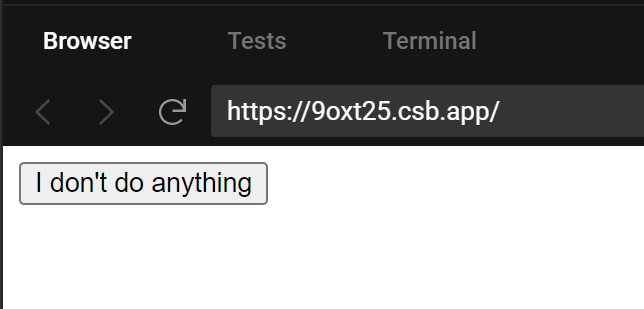
The code looks like this
export default function Button() {
function handleClick() {
alert('Button Clicked');
}
return (
<button onClick={handleClick}>
Click me
</button>
);
}
the handleClick
is an event handler because it handles an event that is the click of a button
Important things to note about event handlers
- Event handlers are defined inside your components
- Event handlers have names that start with Handle and after that the name of the event they are handling in this case it is a click event
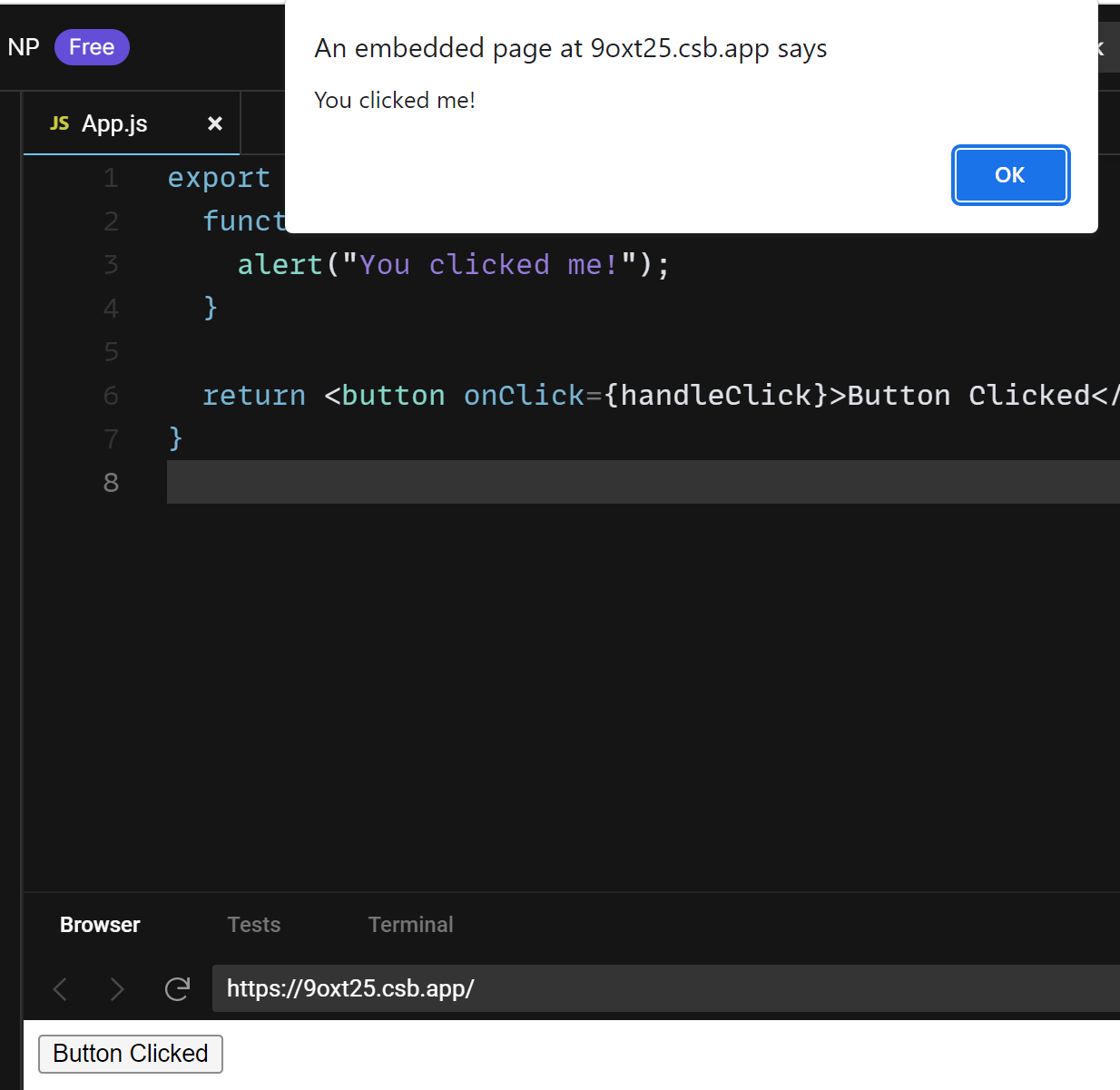
Alternate ways of defining event handlers
Dead Simple Chat allows you to add chat in minutes to your React Application with the powerful JavaScript Chat SDK and API.
- Inline with JSX.
<button onClick={function handleClick() {
alert('button clicked');
}}>
You can also do arrow function
<button onClick={() => {
alert('You clicked me!');
}}>
Inline event handlers are convenient for short functions
Step2: Reading props in event handlers
Dead Simple Chat allows you to add chat in minutes to your React Application with the powerful JavaScript Chat SDK and API.
Because event handlers are declared inside of a component. The event handlers have access to the components props
Let us create a button that shows an alert with the message props form the parent function
function AlertButton({ message, children }) {
return (
<button onClick={() => alert(message)}>
{children}
</button>
);
}
export default function Toolbar() {
return (
<div>
<AlertButton message="cool thing button clicked!">
Cool Thing
</AlertButton>
<AlertButton message="Awesome button clicked!">
Awesome
</AlertButton>
</div>
);
}
These two buttons show different messages when clicked. The name of the buttons as well as the message is passed as props to the AlertButton
function.
You can try changing the message and the message will update in real time.
Step3: Passing Event Handlers as Props
Dead Simple Chat allows you to add chat in minutes to your React Application with the powerful JavaScript Chat SDK and API.
You can pass an event handler as a prop to the component.
Like for example a parent component might pass the event handler as a prop to the child component. Consider a button component, depending on where the button is you might want to execute a different function
At one place that button needs to call a function to play a movie, at another place it might have to call a function that uploads an image
function Button({ onClick, children }) {
return (
<button onClick={onClick}>
{children}
</button>
);
}
function PlayButton({ movieName }) {
function handlePlayClick() {
alert(`Playing ${movieName}!`);
}
return (
<Button onClick={handlePlayClick}>
Play "{movieName}"
</Button>
);
}
function UploadButton() {
return (
<Button onClick={() => alert('Uploading!')}>
Upload Image
</Button>
);
}
export default function Toolbar() {
return (
<div>
<PlayButton movieName="tech movie" />
<UploadButton />
</div>
);
}
Here we have a Toolbar function that renders two button PlayButton and UploadButton
- PlayButton passes handlePlayClick as the onClick to the Button inside of the playclick function
- Upload function passes the function ()=> alert(uploading!) as the onClick prop to the Button
Lastly the Button component accepts a prop called onClick it passes the prop to the browsers built in <button> and onClick={onClick}
This tells the react to call the passes function when the user clicks
In a design system the components like PlayButton
and UploadButton
will pass handlers down and components like button usually contain styling components.
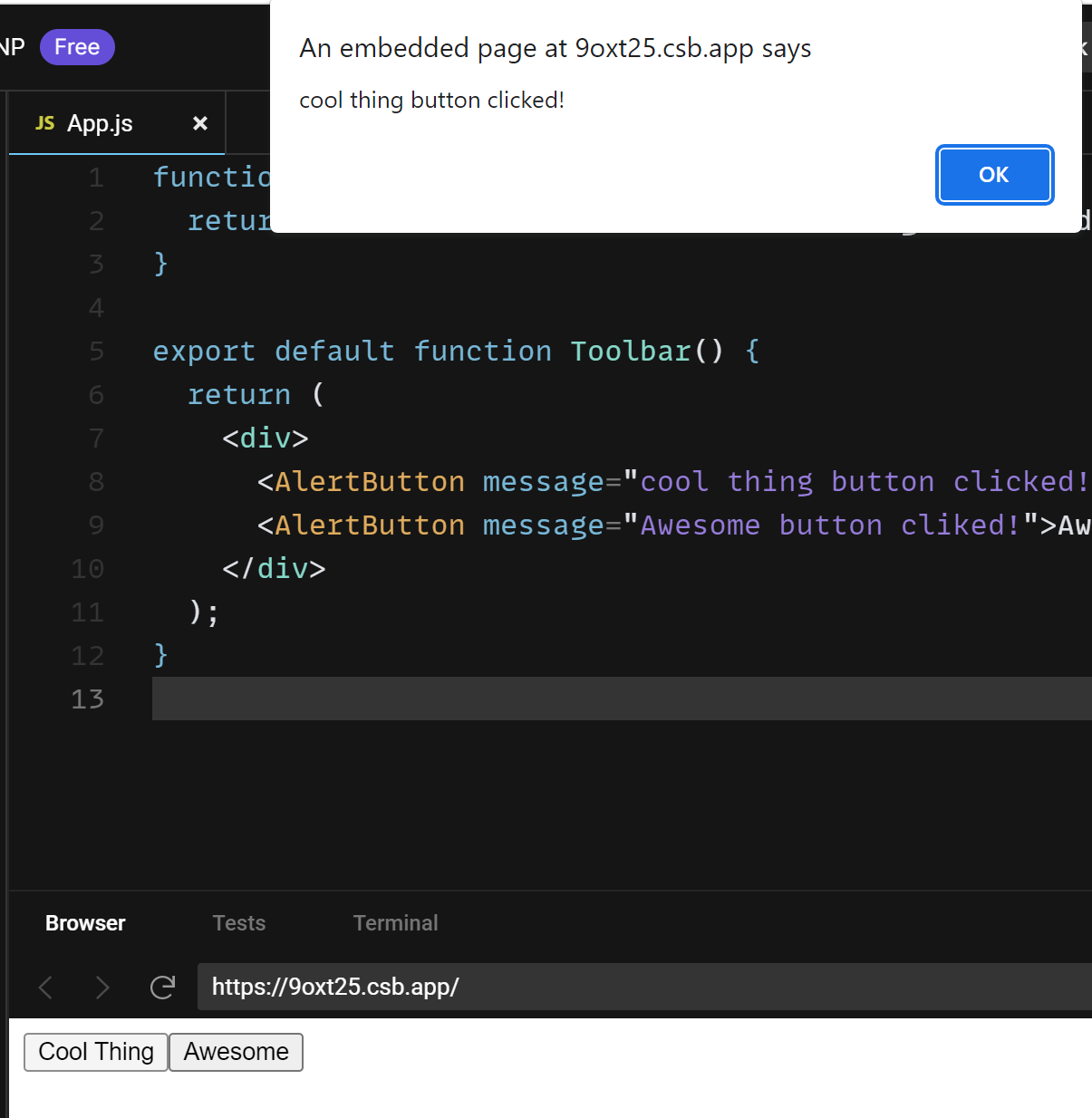
Step4: Naming event handler props
Dead Simple Chat allows you to add chat in minutes to your React Application with the powerful JavaScript Chat SDK and API.
Built in components like <button> and <div> only accept browser event names like onClick
but if you are creating your own components then you can name their event handler any name you like
By convention an event handler name should start with an on and a capital letter for the next word
for example the button onClick
prop could also be called onPress
function Button({ onSmash, children }) {
return (
<button onClick={onPress}>
{children}
</button>
);
}
export default function App() {
return (
<div>
<Button onPress={() => alert('button Pressed 1!')}>
Play Movie
</Button>
<Button onPress={() => alert('Buttonm Pressed 2!')}>
Upload Image
</Button>
</div>
);
}
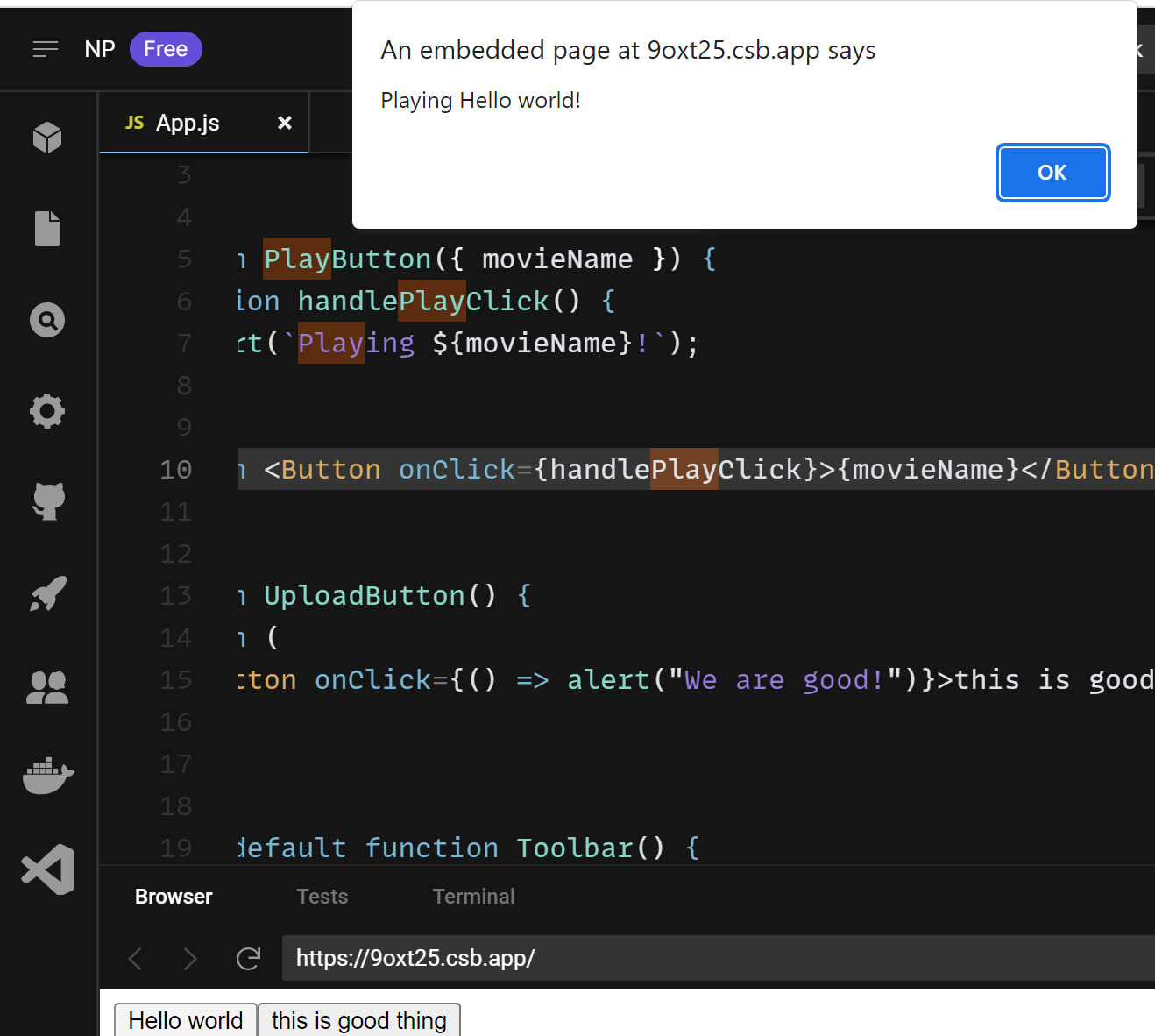
Step5: Event Propagation
Dead Simple Chat allows you to add chat in minutes to your React Application with the powerful JavaScript Chat SDK and API.
Event handlers will watch events from any children the component might have. We say an event bubble or propogates up the tree.
It starts with where the event happened and then goes up the tree
For example, let us consider the code below. It has a div and 2 buttons inside the div
When any of the button is clicked the onClick function is called first but then as the event propogates upwards the toolbar onclick function is also called
export default function Toolbar() {
return (
<div className="Toolbar" onClick={() => {
alert('You clicked on the toolbar!');
}}>
<button onClick={() => alert('Playing!')}>
Play Movie
</button>
<button onClick={() => alert('Uploading!')}>
Upload Image
</button>
</div>
);
}
And if you clicked tool bar itself the only toolbar onclick will be called and hence only one alert function will be called
Step5: Stopping propagation
Event handler receive only the event object as an argument. By convention it is called an e and it stands for event
You can read the event information from the event object.
This event object also lets you stop the bubbling or the propagation
If you call the e.stopPropogation()
the propagation stops
for example:
function Button({ onClick, children }) {
return (
<button onClick={e => {
e.stopPropagation();
onClick();
}}>
{children}
</button>
);
}
export default function Toolbar() {
return (
<div className="Toolbar" onClick={() => {
alert('You clicked on the toolbar!');
}}>
<Button onClick={() => alert('Playing!')}>
Play Movie
</Button>
<Button onClick={() => alert('Uploading!')}>
Upload Image
</Button>
</div>
);
}
When you click on the button for the playMovie or the UploadImage. The react calls the onClick
handler that is passed to the button but the handler defined in the Button does the following
Calls e.stopPropogation()
preventing the event from bubbling further
calls the onClick
function which is a porp
passed from the toolbar function
That function which is defined in the toolbar calls the toolbars own alert function but that is stopped propagating
from the e.stoppropagation
function
Clicking on the button shows a single alert from the button rather than 2 alerts from the button and from the toolbar
Clicking a button is not the same as clicking a totals and so stopping the propagation makes the sense for the UI
Step6: Alternative to propagation: Passing Handlers directly as props
Notice the clickHandler
runs a like of code and then calls the onClick
prop passed by the parent
function Button({ onClick, children }) {
return (
<button onClick={e => {
e.stopPropagation();
onClick();
}}>
{children}
</button>
);
}
You can add more code to this handler before calling the parent onClick
event handler
This pattern is an alternative to propagation
By this way the child component handles the event and the parent components get to specify some additional behavior
The benefit of this pattern is that you can clearly follow the whole chain of event
but unlike propagation this is not automatic
Step7: Preventing default behavior
some browser events like the from submit event have default behaviour built into them when the submit button is clicked the whole page reloads by default
export default function Signup() {
return (
<form onSubmit={() => alert('Submitting!')}>
<input />
<button>Send</button>
</form>
);
}
you can call e.preventDefault()
on any event to prevent this from happening
Both the e.preventDefault
and e.stopPropogation
are useful but different methods
the e.stopPropogation
stops the event handlers that are attached to the browser tags from propogating
the e.preventDefault
stops the default behaviour of the html tags from happing
Step8: Are there any side effects to event handlers
Yes
unlike rendering functions the event handlers do not need to be pure. So theuys are as great place tope change something
Step9: Recap
- You can handle events by passing a function to an element as a prop
- Event handlers must be passed and not called react calls them for us like for example
onClick={handleClick}
and notonClick={handleClick()}
- You can define an event handler function seprately or inline
- event handlers can be defiend in the parent and passed to the child as a prop
- events propogate updawards or bubbles upwards and can be stoped by using the e.stopProppogation method
- Browser elements may have unwanted behaviour call
e.preventDefault()
to prevent that - Explicitly calling an event handler prop from a child handler is a good alternative to propagation