With useDebugValue you can add a label to a custom hook in react dev tools
useDebugValue(value, format?)
let us explore in more detail about the useDebugValue react hook
Using the useDebugValue hook you add a label to any custom hook, which can be see in the react dev tools
This can be very useful in debugging applications with numerous custom hooks
Basically the useDebugValue is used to parse a value next to the custom hook when inspected using the React Dev Tools
you can also format the debug value using an optional method provided by the useDebugValue to improve readability when inspecting components
useDebugValue(value, format?);
Reference
You can call useDebugValue at the top of your custom hook to display a readable debug value
import { useDebugValue } from 'react';
function showOnlineOrNot() {
// ...
useDebugValue(isOnline ? 'Online' : 'Offline');
// ...
}
value
: The value that you want to display on the react dev tools- optional
format
: The format function formats the value into a readable format. If you don't specify the formatting function then orignal value itself will be displayed
Returns
The useDebugValue
does not return anything
Usage
Let us explore how to use the useDebugValue
to debug the value of a
add a label to a custom hook
To add a label to your. custom hook, simply call the useDebugValue
at the top of your custom hook and the label will appear in the React Dev tools
like
import (useDebugValue} from 'react';
function useInternetConnection() {
let isOnline = */code to check if the user is online/*
useDebugValue(isOnline? 'Online': 'Offline');
// ..
}
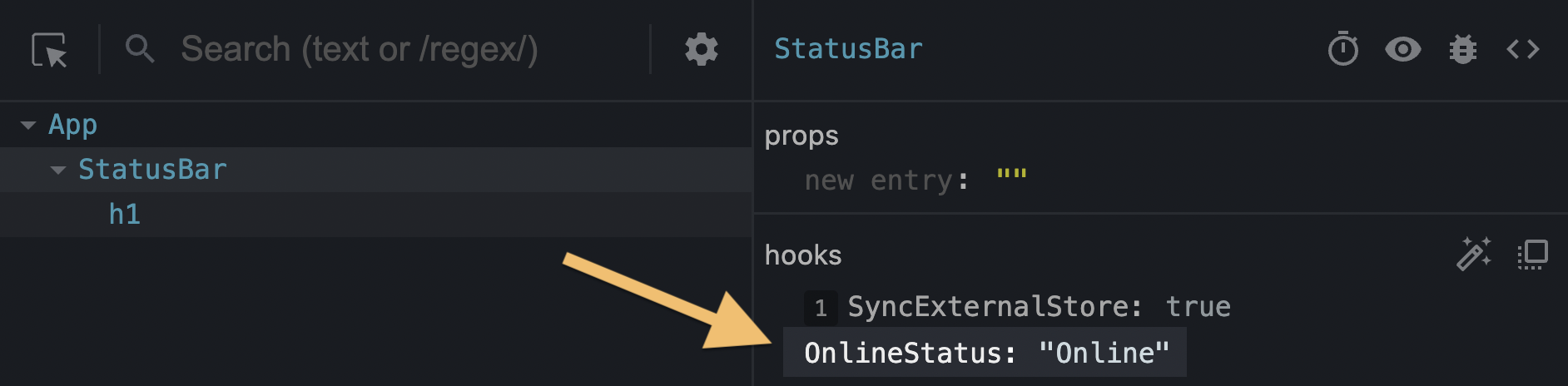
The components calling useInternetConnection
gets a label OnlineStatus: "Online"
when some one inspects them
Without the useDebugValue
only the underlying data that is the value true
will be shown
Deferring formatting of a debug value
If you are using a computationally intensive formatting function, You can optimize the performance of your application by deferring the useDebugValue
to defer the computation until the component is inspected
let us look at a
import { useDebugValue } from 'react';
function useEventDate() {
let eventDate = /* get the event date... */
useDebugValue(eventDate, date => date.toDateString());
return eventDate;
}
As we can observe, In this example the function toDateString
is only called when the element is inspected and the useDebugValue runs
Using useDebugValue with useState
useDebugValue
can be used along with useState
in custom hooks to provide more context about the internal state during debugging
Here is a simple example of useDebugValue
used with useState
import { useState, useDebugValue } from 'react';
function useCounter() {
const [count, setCount] = useState(0);
useDebugValue(count === 0 ? 'Not started' : `Count: ${count}`);
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
return { count, increment, decrement };
}
In this case we are using the useState
to manage the count state. useDebugValue
is used to display the value in react dev tools
Using multiple useDebugValue hooks
Using mutiple useDebugValue hooks in a custom hook is possible Each call to useDebugValue
is a seprate label that is being attached to a hook
let us consider an examples
import { useState, useDebugValue } from 'react';
function useCounter() {
const [count, setCount] = useState(0);
const [isStarted, setIsStarted] = useState(false);
useDebugValue(count === 0 ? 'Not started' : `Count: ${count}`);
useDebugValue(isStarted ? 'Started' : 'Not started');
// Other hook logic...
}
In this example we are using two seprate debug values one for count and one for isStarted
Using Objects and Arrays with useDebugValue
We can use Objects asn well as Array with useDebugValue` let us consider examples on both
import { useDebugValue } from 'react';
function useItems() {
const items = /* Fetch or compute items... */
useDebugValue(`Items count: ${items.length}`);
return items;
}
and we can also use Objects like this
import { useDebugValue } from 'react';
function useObject() {
const obj = /* Fetch or compute object... */
useDebugValue(`Object keys: ${Object.keys(obj).join(', ')}`);
return obj;
}
Performance issues and considerations
It is important to consider performance issues when using useDebugValue
. The`useDebugValue` is lightwieght but over using this could cause performance issues
If you are using a computationally expensive optimizer funtion to parse large amounts of data you can defer it to improve performance and only render it when inspected in dev tools
Conclusion
the useDebugValue
is a beneficial hook in react that enables you to add human readables lables to your hooks
We have demonstrated how to use the useDebugValue
along with examples
I hope that you like the article and thank you for reading