In this article we are going to cover important react concepts and terms try them out in examples.
You will get enough knowledge to get you up and running writing simple apps and ready to learn and understand advanced topics and tutorials
If you are looking for a React Native Chat SDK to build in app chat messaging application, you can consider DeadSimpleChat React Native SDK
What are components?
- Components: Components is a piece of UI (user interface) with their own logic and appearance.
- A Component can be a button or even a full webpage, it depends on how you structure your react application
- there are class and functional components but functional components are preferred by the react and class components are slowly being deprecated
- Let us consider an example of a button component:
function SimpleButton (){
return (
<button>This is a simple button</button>
);
}
We can also nest components within other components. So we can nest the button component inside the App component like so
export default function ReactApp(){
return (
<div>
<h1>This is a React APP</h1>
<SimpleButton />
</div>
);
}
React component names must start with a capital letter that is why the SimpleButton
starts with a capital letter and the normal HTML tags start with small letters.
Here is what the complete application looks like
function SimpleButton (){
return (
<button>This is a simple button</button>
);
}
export default function ReactApp(){
return (
<div>
<h1>This is a React APP</h1>
<SimpleButton />
</div>
);
}
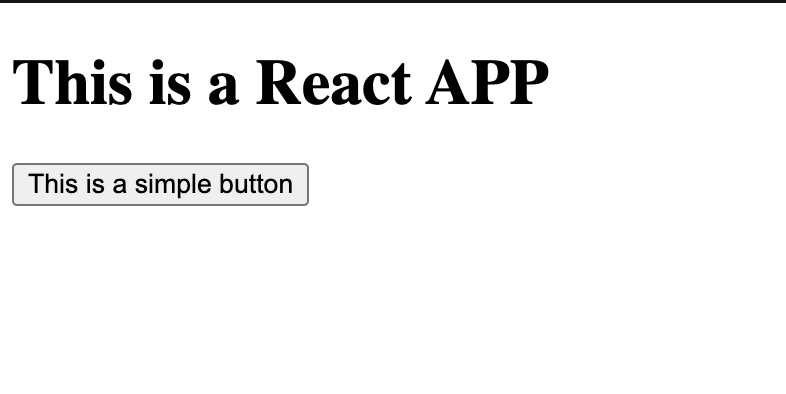
The export default
specify which is the main component in the file that will be exported for outside the file.
Jsx : An Introduction
JSX is like HTML but stricter, in the sence that syntax needs to be correct
for example you need to close the tags like <br />
you cannot leave them open. The react will give an error
Also, you can not return multiple JSX tags in the return statement above
Only one tag can be returned, so if you have multiple tags you can wrap them in these
function HelloWorld() {
return (
<>
<h1>hello world</h1>
<p>Cool</p>
</>
);
}
There are many HTML to JSX converters available online that you can use to convert HTML to JSX
Showing data on the Screen
You can write JavaScript inside of the JSX. You can write JavaScript code within the curly braces.
So, for example if you have a variable somewhere in your code that has data that you wish to show on screen,
you can do that like
return (
<h1>
{product.name}
{product.quantity}
</h1>
);
One thing to remember is that the react processes the javascript and then returns the result of the code to the JSX
for example
return (
<img
className="product-image"
src={product.imageUrl}
/>
);
the react reads the value of product.imageUrl
and then passes it to the JSX
Conditional Rendering
You can use your normal javascript if
and while
statements to render code conditionally.
You basically use your normal javascript rendering to conditionally render the code
here is an example
let webPage;
if (isLoggedIn) {
webPage = <Dashboard />;
} else {
webPage = <loginPage />;
}
return (
<div>
{webPage}
</div>
);
Render Lists
In React you can easily render lists, you can use for
loops or array map()
function to render lists of components
const products = [
{title: 'Apple', id:1 },
{title: 'Sunbeam', id:1 },
{title: 'Mouse', id:1 },
{title: 'PineApple', id:1 },
];
Here we can use the map()
function to convert an array of products in to an array of <li>
items:
const listItems = products.map(product =>
<li key={product.id}>
{product.title}
</li>
);
return (
<ul>{listItems}</ul>
);
Notice the key
attribute. For each item on the list we need to specify a unique string or number that identifies the item
React uses the key to determine what happens if you later insert, delete or reorder items
Responding to events
to respond to events like a click of a button, mouse scroll, focus or any other event. You need to declare event handler functions inside the component
These are functions inside your functional component that gets called when the event happens (event that you need to respond to )
for example
function AlertButton() {
function handleClick() {
alert("the button was clicked");
}
return <button onClick={handleClick}>click the button</button>;
}
Notice that there are no parantheses at the end of onClick={handleCLick}
that is because you need to pass the event handler function on the onClick not call the event handler function on onClick
React will call the event handler function when the user click the button
Remembering the data
Sometimes, you want the component to remember some information and display it.
You can store information in the state and then retrive it when you need it. For example you might need to store how many time a button might have been click or how many people are there in a chat
To remember stuff you need to import useState
from react
import {useState} from 'react'
Now you can declare a state variable inside your component
import { useState } from "react";
function AlertButton() {
const [count, setCount] = useState(0);
//...
}
Here we have declared the state to 0. So when the component is first rendered the count state is 0.
We can certainly change the state, to change the state we do not directly change it. instead we call the setState function here the setCount function and then we pass the value to it
here is how its done
import "./styles.css";
import { useState } from "react";
function ClickCounter() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1);
}
return <button onClick={handleClick}>Button Clicked {count} times</button>;
}
export default function ReactApp() {
return (
<div>
<h1>This is a React APP</h1>
<ClickCounter />
</div>
);
}
Here when we call the handleClick function the state count is incremented by 1. Then react will render the component function again and so on
Note: If you render the component multiple times each will get its own state
for example
import "./styles.css";
import { useState } from "react";
function ClickCounter() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1);
}
return <button onClick={handleClick}>Button Clicked {count} times</button>;
}
export default function ReactApp() {
return (
<div>
<h1>This is a React APP</h1>
<ClickCounter />
<br />
<ClickCounter />
</div>
);
}
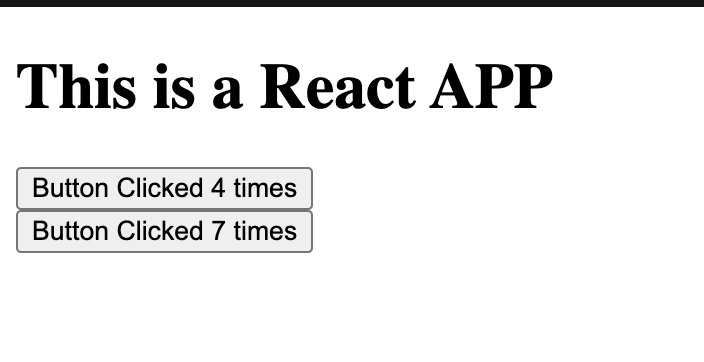
Here each button individually remembers its count and does not affect other buttons
Hooks: an introduction
functions that start with use
are called as hooks. We have already used one such hook called the useState
There are a lot of built in hooks that you can look up in the react api reference
You can also write your own hooks by combining one or more existing hooks.
Note: You can only call hooks at the top of your component or (on top of other hooks)
Sharing data between components (lifting the state)
In the above example of a button. The each ClickCounter button had its own state and data
But what if we wanted both the buttons to share the data. For example if we want the count to increase in both the buttons no matter which button is clicked
To do this we need to move the state up. That is if we want both the buttons to update the count at the same time then we need to move the state from both these components to a parent component that is the closest component containing both these components
let us see the example
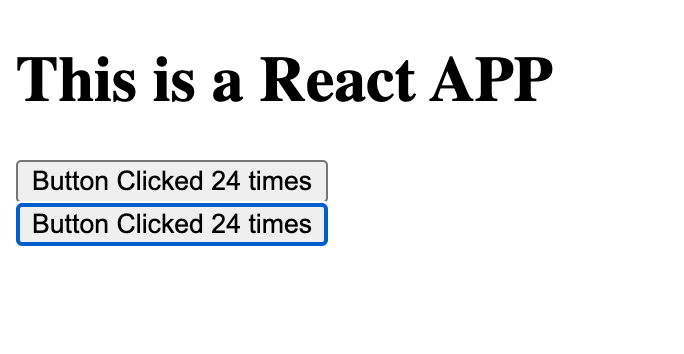
import "./styles.css";
import { useState } from "react";
function ClickCounter({ count, onClick }) {
return <button onClick={onClick}>Button Clicked {count} times</button>;
}
export default function ReactApp() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1);
}
return (
<div>
<h1>This is a React APP</h1>
<ClickCounter count={count} onClick={handleClick} />
<br />
<ClickCounter count={count} onClick={handleClick} />
</div>
);
}
What are we doing here:
- We are moving the state from individual
ClickCounter
components to the component that is just above them which is theReactApp()
component
2. We imported the useState from react
3. then we moved the state from the ClickCounter
component to the component that was just above it that is the ReactApp
component
4. We also moved the handleClick()
from the ClickCounter
to the ReactApp component
5. then we are returning the ClickCounter
function there you can see the count and onClick are passed to the ClickCounter
as function
6. You can pass the props to the react just like you pass parameters to a function.
7. we are passing the count and handleClick function as props to the ClickCounter
8. Then ClickCounter
returns the button with the onCLick and count parameters
9. Now when the button is clicked the data is coming from the useState
that is in the ReactApp and not in the ClickCounter so both the buttons update when even one is clicked
Conclusion
In this article I have given you a quick overview of react and how it works with the help of examples
I hope the article helped you. Thank you for reading article