Editor’s note:This article was last updated on 6 July 2024 to include the addition of the New ES6+ Features for converting strings to numbers
Type conversion in JavaScript, also called as type casting is a process of converting a value of one type (String) to another type (Number).
This process is an essential part of writing code in JS because JavsaScript is a loosely typed language where var
do not have a fixed type and can be assigned and re-assigned any type
Categories of Type Conversion in JS
- Explicit Type conversion also called as type casting: When a developer intentionally converts one type into another using functions or contructs provided by the programming language for this purpose. It is called as explicit type conversion. Example in JavaScript using the
Number()
function - Implicit Type Conversion: JavaScript programming language also does type conversion automatically based on the context of the operation. This is called as implicit type conversion as the developer is not explicitly type converting the value. Using the
==
operator to compare a String and a Number, it is an example of implicit type conversion
Traditional methods to convert String to Number in JavaScript
parseInt()
andparseFloat()
functions- Unary plus
+
operator
parseInt()
and parseFloat()
functions
1. parseInt()
Syntax: parseInt(string, radix)
string
: The value of string that needs to be parsed or converted into a number. The function expects the value to be a string if it not a string than it is automatically converted into string using thetoString()
methodradix
an optional integer between the range of 2 and 36 that represents the root or the base of the numerical system. If nothing is provided by default 10 is used.
parseInt()
is useful when you want to parse a string and convert it into an integer. It is mmore useful when you want to convert strings with different bases such as binary, octal or hexadecimal
If you are looking for a JavaScript chat SDK to build chat messaging, you can consider DeadSimpleChat JavaScript SDK
Practical example
let input = "100px";
let number = parseInt(inputString, 10); // number will be 100
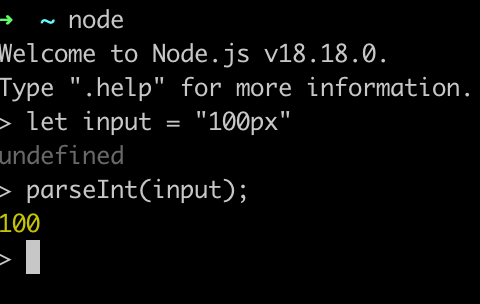
Handling White Spaces, Char and special cases
- Whitespace: leading whitespace in the string is ignored
- Char: If the first char of the string cannot be converted into number the parseInt() function returns a NaN, after that if there is a string that cannot be converted into a number it ignores the rest of the string
2. ParseFloat(string)
Syntax parseFloat(string)
Parameters:
- string: The value to parse. If the value is not a string it be automatically converted into a string
Practical example
let inputString = "100Something";
let number = parseFloat(inputString); // number will be 100
Handling white spaces, char and special cases
- Whitespace: Leading whitespace is ignored
- char: Parsing is continued until the function reaches a char that cannot be converted into a number. If it is the first char than the function returns a NaN
- Special Cases: There is no
redix
parameter.
3. Urinary +
Operator
This is a shorthand method for converting strings to number in javascript
here is how you can do this
let stringNumber = "123";
let convertedNumber = +stringNumber; // convertedNumber is now the number 123
While it is an easy way to type convert string to number it does comes with its pitfalls here are some
- Leading Zeros: Unlike parseInt() if you give it something other than integers, it might not work properly.
- Non-Numeric Strings: If the string is not a number the resultant would be NaN
- Implicit Coercion Risks: unary plus can often leads to errors when it is not clear when an operation requires type conversion or not. These types of operations and risks can be mitigated when using explicit type conversion methods
Modern Techniques for type conversion from string to number
The number function was released in the new ECMAScript language standared and it provides a more general purpose conversion than parseInt()
or parseFloat()
functions
Syntax
4. Number(value)
value: The value that needs to be converted into a numberic type. This value can be Boolean, String or an Object
The number()
function returns a primitive value converted or a integer or a floating point number.
Here are some of the real life examples of using the Number() function to convert strings to number
Number('123'); // 123
Number('12.3'); // 12.3
Number('123e-1'); // 12.3, exponential notation
Number(''); // 0, empty string
Number('0x11'); // 17, hexadecimal
Number('0b11'); // 3, binary
Number('0o11'); // 9, octal
Number('foo'); // NaN, non-numeric string
Number('100a'); // NaN, invalid numeric string
Number(true); // 1
Number(false); // 0
Number(null); // 0
Number(undefined); // NaN
Number({ valueOf: () => '123' }); // 123, object with valueOf method
Detailed Behaviour:
- Boolean:
true
is converted to 1 and false is converted to 0 - Null: Null is converted to 0
- undefined: undefined is converted to
NaN
- String:
- WhiteSpaces: White Spaces at the beginning and at the end of the string are ignored
- If the String is empty the returned value is 0
- Strings correspondin to binary and octal types are supported
- If a string does not represent a valid number then the function returns NaN
- Objects: the
Number()
function first calls thevalueOf()
method if that returns a value it converts it into a number. If not then the Number function tries calling the toString() method to convert the Object to a string and then tries to convert that string to a number
5. Math.floor()
and Math.round()
Javascript offers various Math functions these functions can be used for converting floating point numbers to integers but also can be used for type conversion
let us look at some of the Math functions and how they can be used for type conversion
math.floor()
// Convert string to number and round down
const stringInput = '5.8';
const numericFloor = Math.floor(stringInput); // Converts '5.8' to 5
console.log(numericFloor); // Outputs: 5
However using Math functions to convert strings to numbers is not recommended because the Math functions expects Number type and JavaScript first tries to implicitly type convert string to number before passing the value to the Math function
Bonus: Using Libraries for type conversion
You can also use libraries to do type conversion. People use this method for various purposes like
- Consistency: It is company policy to use library features so as to maintain consistancy
- Simplicity: Many devs are accustomed to using a specific library and hence use it
- Enhanced features: The libraries might support enhanced features and error handling than traditional JavaScript functions
Example: Lodash
Lodash is a popular javascript utility library that provides consistant behaviour and performance improvement over traditional JS
Here is how you can use Lodash for string to number conversion
const _ = require('lodash');
let aNumber = _.toNumber('43'); // Converting the string to number, result is 43
let floatNumber = _.toNumber('3.1'); // Converting the string to floating-point number, result is 3.1
let notANumber = _.toNumber('abc'); // Cannot be converted, result is NaN
Angular
In angular filters are used to format the data. For string to number conversion, the logic is typically handled within components or services using typescript which provides a robust type system
let us look at an example
// Using a Angular service or component
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class NumberConversionService {
constructor() { }
convertToNumber(value: string): number | null {
let parsed = parseFloat(value);
return isNaN(parsed) ? null : parsed;
}
Best Practices and Common Pitfalls
Error handling and Validation
try/catch:
Use the try/catch
blacks when dealing with risky conversions that might throw errors. This can be done like
try {
let parsedNumber = JSON.parse(someInput);
} catch (error) {
console.error('Parsing error:', error);
}
isNaN
check if the value is NaN
which is a possible result when the conversion fails.
let num = Number(someString);
if (isNaN(num)) {
console.log('The NaN is a result of the conversion');
}
isFinite:
This function checks if the number is finite or not. It returns infinity
or NaN
if the number is not finite
Copy code
let num = Number(someString);
if (!isFinite(num)) {
console.log('The number is infinite');
}
Practical Examples and Use Cases
In this section we are going to look at interactive examples of different use-cases
- Simple Conversion
let someValue = "234";
console.log(Number(someValue)); // result: 234
console.log(+someValue); // result: 234
console.log(parseInt(someValue)); // result: 234
2. Floating Point Conversion:
let floatingPointString = "678.2343";
console.log(parseFloat(floatingPointString)); // result: 678.2343
3. Binary, Hexadecimal and Octal Conversion:
let binaryString = "1010";
let hexString = "1F";
let octalString = "10";
console.log(parseInt(binaryString, 2)); // result: 10
console.log(parseInt(hexString, 16)); // result: 31
console.log(parseInt(octalString, 8)); // result: 8
4. Form Input Parsing
When building web forms the input are many times recieved as string and then need to be converted into numbers if we need to perform some calculations
function calculateTotal(price, quantity) {
return Number(price) * Number(quantity);
}
let priceInput = "12.99";
let quantityInput = "6";
console.log(calculateTotal(priceInput, quantityInput)); // result: 77.94
5. Query String parsing
URL parameters are string but in many usecases you might want to convert them into integers
You can do this like so
function getQueryParam(param) {
let params = new URLSearchParams(window.location.search);
return params.get(param);
}
let page = getQueryParam("page");
console.log(typeof page); // result: "string"
page = parseInt(page) || 1;
console.log(typeof page); // result: "number"
These are some of the examples of parsing string to numbers in javascript
New ES6+ Features for converting stringd to numbers
Template Literals
Template litrals are useful when you are dynamically creating strings from multiple variables
You can use template litrals for formatting strings that need to be converted into numbers
Ensuring that strings are correctly formatted before converting them to numbers is useful in formatting outputs from various scources to number ready data
const value = '100';
const formattedNumber = `${value}.00`; // Ensuring the format must include decimal places
const number = parseFloat(formattedNumber);
console.log(number); // result: 100
Arrow Functions
Arrow functions are useful for converting string to numbers in line.
This is particularly important in array transformations or other functional programming patterns
for example you can map an array of string numbers to actual strings using the arrow functions
const stringNumbers = ['1.1', '2.2', '3.3'];
const numbers = stringNumbers.map(number => parseFloat(number));
console.log(numbers); // result: [1.1, 2.2, 3.3]
Destructuring Assignment
Using destructuring you can extract string values from arrays and objects, that need to be converted into numbers
for example
const data = { strNumber: '1234' };
const { strNumber } = data;
const number = Number(strNumber);
console.log(number); // result: 1234
Default Parameters
Using default numbers make sure that program does not crash by providing a feedback number when input are missing or undefined
Using a default values makes sure that string to number conversion always have valid data
function convertToNumber(str = '0') {
return Number(str);
}
console.log(convertToNumber('123')); // results: 123
console.log(convertToNumber()); // results: 0, using default parameter
New Number Methods
The ES6 introduced the Number.isNaN()
and the Number.isFinite()
methods, these functions are more reliable than the previous isNaN()
and isFinite()
methods because these methods do not force their arguments to be numbers
const result = Number('NaN');
console.log(Number.isNaN(result)); // true
const validNumber = Number('123');
console.log(Number.isFinite(validNumber)); // true
BigInt
when you are handling very large or very small numbers that are out of the safe range of standard javascript.
The BigInt is a newer addtion in ES6 that can work for you
const largeNumber = '8732643265328648723';
const bigIntNumber = BigInt(largeNumber);
console.log(bigIntNumber); // results: 8732643265328648723
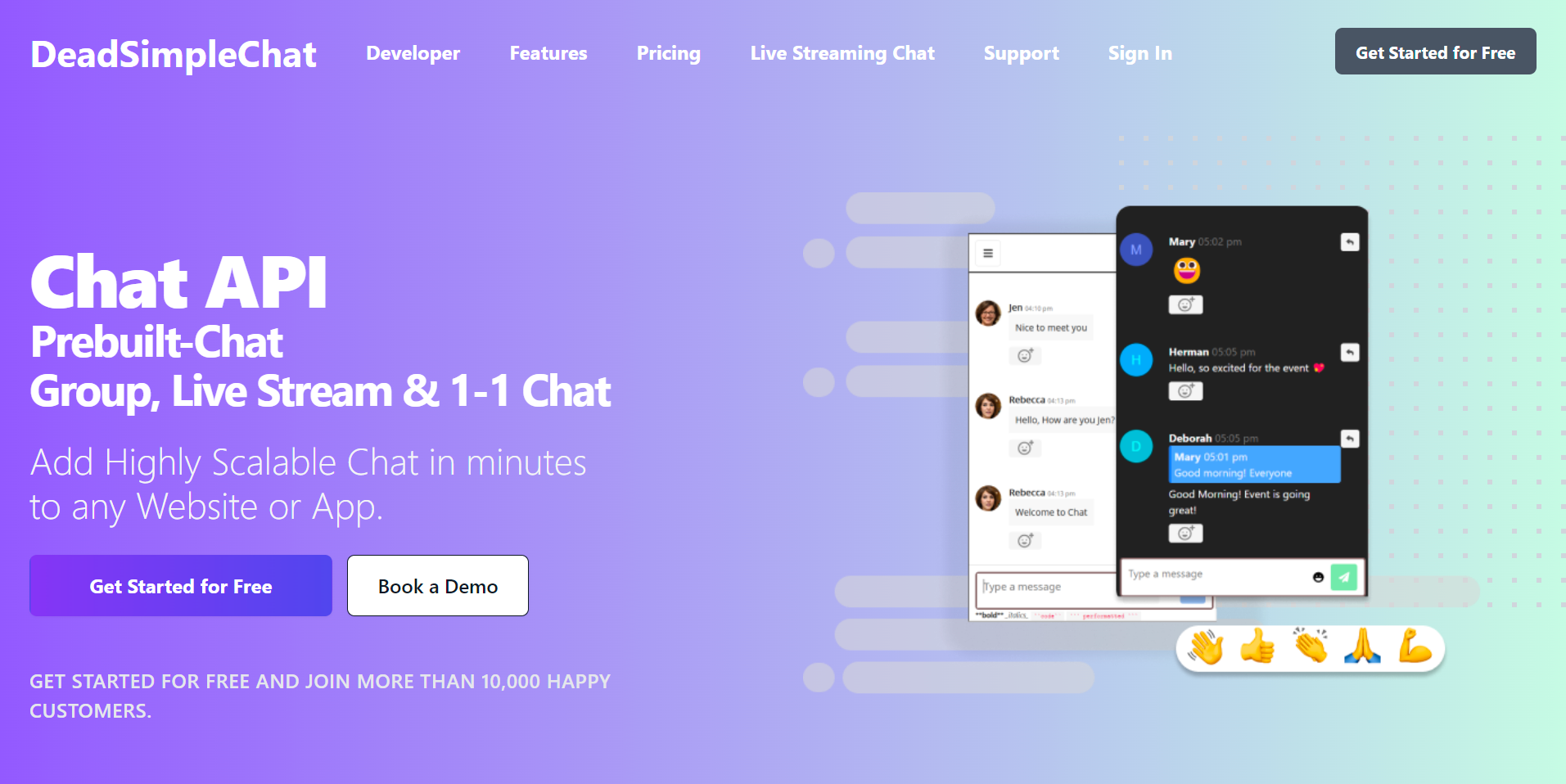
Need Chat API for your website or app
DeadSimpleChat is an Chat API provider
- Add Scalable Chat to your app in minutes
- 10 Million Online Concurrent users
- 99.999% Uptime
- Moderation features
- 1-1 Chat
- Group Chat
- Fully Customizable
- Chat API and SDK
- Pre-Built Chat
Need TURN server, we recommend going with Metered TURN servers starting at 0.04 USD / GB
If you need a free version of the turn server we also have the OpenRelayProject.org a free TURN server.